Dependency Matrix tool allows templates to access data from a Dependency Matrix, use diagrams to get data, row elements, column names, or relations between row and column elements from the matrix.
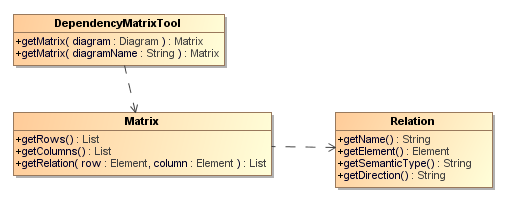
A Class diagram of the DependencyMatrixTool.
You can use the following Dependency Matrix tool API in the template.
Class DependencyMatrixTool
Use the following methods to use diagrams to get data.
- +getMatrix( diagram : Diagram) : Matrix
- +getMatrix( diagramName : String) : Matrix
Class Matrix
Use the following methods to use diagrams to get row elements, column elements, and relations between row and column elements.
- +getRows() : List<Element>
- +getColumns() : List<Element>
- +getRelation( row: Element, column : Element) : List<Relation>
Class Relation
Use the following methods for class relations.
- +getName() : String
- +getElement() : Element
- +getSemanticType() : String
- +getDirection() : String
Using Diagrams to Get Data from Dependency Matrix
A Dependency Matrix is a special diagram. You can retrieve it using the $Diagram variable. Thus, every method must accept a diagram instance or a diagram’s name. Use the following methods to return a Dependency Matrix instance. You can retrieve rows and columns from the Dependency Matrix instances.
Getting Dependency Matrix Instances from Diagram Elements
getMatrix(diagram : Diagram) : Matrix
To get a Dependency Matrix instance from a specified diagram element, use the following code.
#set($matrix = $depmatrix.getMatrix($diagram))
Where the parameter is:
- diagram – a diagram element
Return an instance of Dependency Matrix.
For example:
#foreach($diagram in $project.getDiagrams("Dependency Matrix")) #set($matrix = $depmatrix.getMatrix($diagram)) #end
Getting Dependency Matrix Instances from Diagram Names
getMatrix(diagramName : String) : Matrix
To get a Dependency Matrix instance from a specified diagram’s name.
#set($matrix = $depmatrix.getMatrix($diagram))
Where the parameter is:
- diagram – a diagram’s name
Return a Dependency Matrix instance.
Example code:
#set($diagram = "diagram name") #set($matrix = $depmatrix.getMatrix($diagram))
Getting Row Elements
The Matrix consists of rows and columns.
Getting All Row Elements
getRows() : List<Element>
Use this method to retrieve a list of row elements.
$matrix.getRows()
The returned value is a list of Elements.
To print all row elements' names, for example, type the following code:
#foreach($row in $matrix.rows) $row.name #end
Getting Column Elements
The matrix consists of rows and columns.
Getting all column elements
getColumns() : List<Element>
Use this method to retrieve a list of column elements.
$matrix.getColumns()
The returned value is a list of Elements.
To print all column elements' names, for example, type the following code:
#foreach($col in $matrix.columns) $col.name #end
Getting Relations between Row and Column Elements
getRelation(row : Element, column : Element) : List<Relation>
Use this method to retrieve the relations between row and column elements.
$matrix.getRelation($row, $column)
Where the parameter is:
- row – a row element
- column – a column element
The returned value is a list of Relations.
The Relation class contains the following methods:
- getSemanticType : String
Return the semantic type - getElement : Element
Return a relationship element or null if the relationship is not an element, for example, tag name. - getName : String
Return a relationship name. If the relationship is a tag name, it will return the tag name. If the relationship is NamedElement, it will return the element name; otherwise, return a human name. - getDirection : String
Return the direction.
To print the row, column, and its relationship name, for example, type the following code:
#foreach($row in $matrix.rows) #foreach($col in $matrix.columns) #foreach($rel in $matrix.getRelation($row, $col)) $row.name has $rel.name with $col.name #end #end #end
To print rows, columns, and relations in a spreadsheet file format, for example, type the following code:
#import('depmatrix','com.nomagic.reportwizard.tools.DependencyMatrixTool') #forpage ($diagram in $report.filterDiagram($Diagram,["Dependency Matrix"])) #set($matrix=$depmatrix.getMatrix($diagram)) $bookmark.create($diagram.name) | ||
#forcol ($col in $matrix.columns)$col.name#endcol | ||
#forrow($row in $matrix.rows)$row.name | #forcol ($col in $matrix.columns)#if(!$report.isEmpty($matrix.getRelation ($row,$col)))-> #end#endcol | #endrow |
#endpage |